Gestures and Input
You can easily let players pinch and drag objects in the world.
Receiving Taps
To receive taps (gaze + a pinch in VisionOS) you do exactly the same thing you do in Godot to receive a mouse click on a Node--you set the input_ray_pickable
property of your CollisionObject3D
to true.
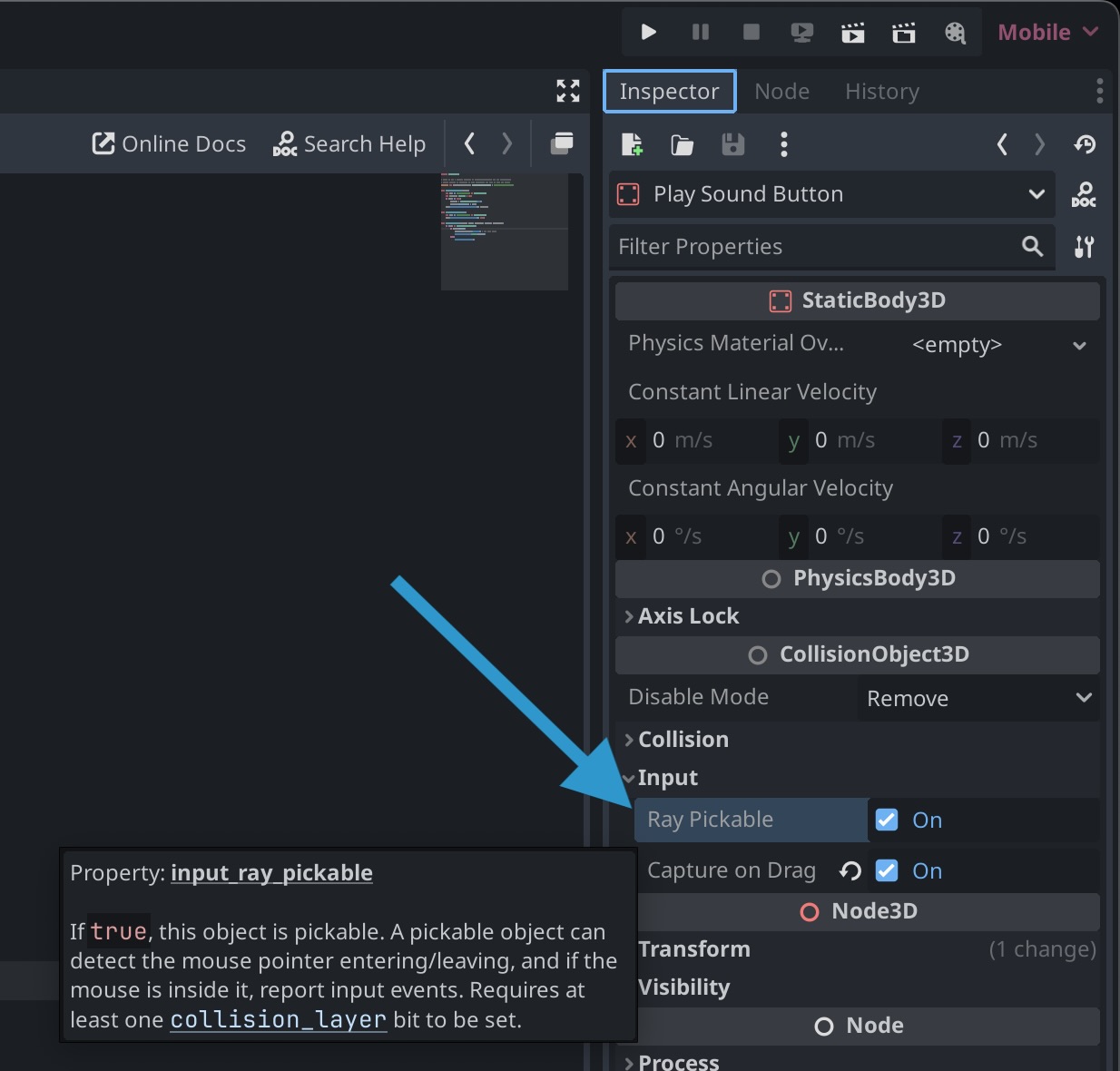
And then connect the input_event
signal to some code that responds to a InputEventMouseButton
:
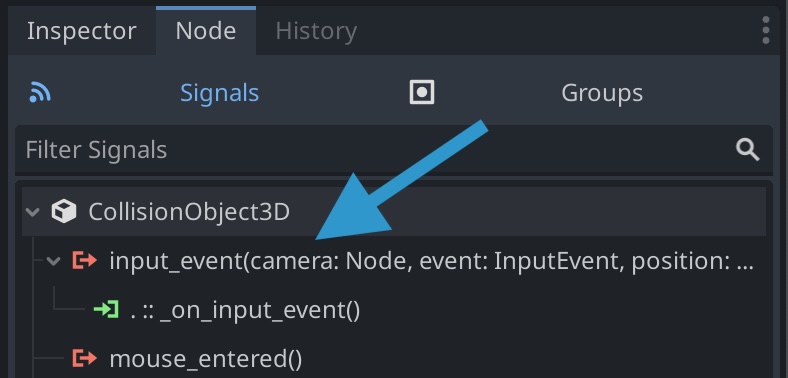
That signal needs to connect to a function in a gdscript. An example script might look like:
extends StaticBody3D
func _on_input_event(_camera, event, _position, _normal, _shape_idx):
if event is InputEventMouseButton:
if event.pressed:
print("TAPPED ON ", self)
How taps map to RealityKit
GodotVision sees CollisionObject3D
s with input_ray_pickable
and adds an InputTargetComponent
to its corresponding Entity.
You can also get a HoverEffectComponent
with metadata hover_effect
--see Metadata for more info.
Special Custom Signals
spatial_drag
signal
Any CollisionObject3D
with a custom signal named spatial_drag
will receive drag gesture events from GodotVision. The signal gets called with a Dictionary with the following parameters:
{
"phase": String, // "began", "changed", or "ended"
"global_transform": Transform3D, // the current Transform3D of the Node
"start_global_transform": Transform3D, // the Transform3D of the Node when the gesture began
}
The GodotVision
addon in res://addons
also has special functionality to simulate the spatial_drag
signal on normal Godot runs of your game, so you can test the interactions with a mouse.
Example Drag Script
Below is a simple version of a "drag" gdscript from the GodotVisionExample "hello" scene. If you attach this to a CollisionObject3D
(like an Area3D
or a AnimatableBody3D
or a StaticBody3D
), you can gaze at the object, pinch, and move your hand to translate and rotate it!
extends Node
signal spatial_drag(Dictionary)
func _ready():
spatial_drag.connect(on_spatial_drag)
func _exit_tree():
spatial_drag.disconnect(on_spatial_drag)
func on_spatial_drag(params: Dictionary):
self.global_transform = params['global_transform'] # Move in 3D with the spatial gesture.
You must configure the CollisionObject3D
with a shape correctly for Godot (and GodotVision) to receive mouse clicks or gaze interactions with it. Godot will warn you if it's not setup correctly:
You can add a child CollisionShape3D
node to give it a shape setup. But then Godot will still warn you that you haven't provided an actual shape resource:
With the CollisionShape3D
selected, you can add a Shape
in the dropdown in the inspector on the right side of the screen:
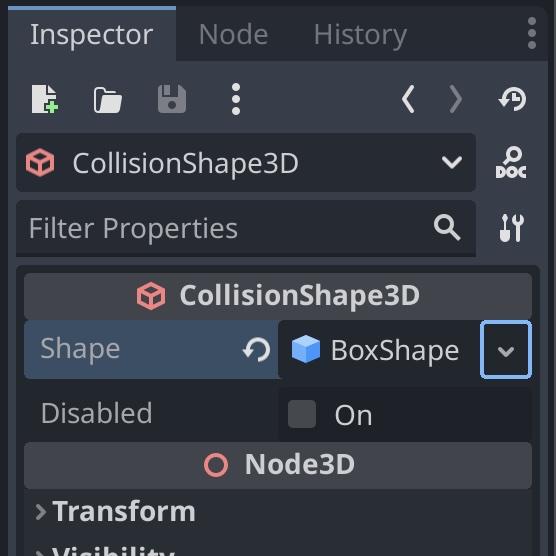
That shape will define the interactable area of your object.